The Data in an Array Can Be Sorted in Either Ascending or Descending Order.
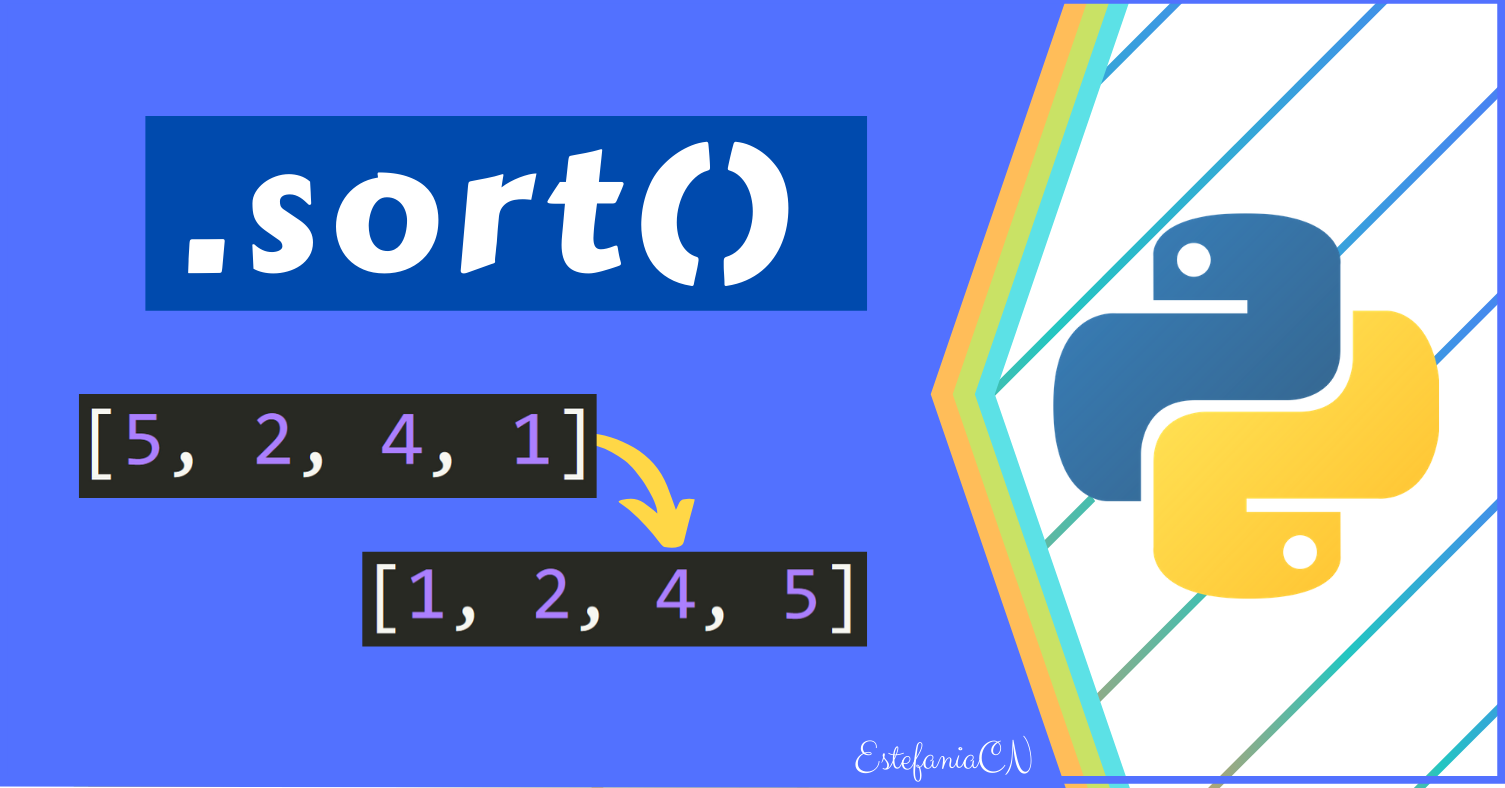
If you desire to learn how to work with the sort()
method in your Python projects, then this article is for you. This method is very powerful and yous tin can customize it to fit your needs, then allow's see how it works in detail.
You will learn:
- How to use this method and customize its functionality.
- When to use it and when not to use it.
- How to call it passing unlike combinations of arguments.
- How to sort a listing in ascending and descending order.
- How to compare the elements of a listing based on intermediate values.
- How you can pass lambda functions to this method.
- How this method compares to the
sorted()
office. - Why the
sort()
method performs a stable sort. - How the process of mutation works backside the scenes.
Are yous ready? Permit's begin! ⭐
🔹 Purpose and Use Cases
With the sort()
method, y'all can sort a list in either:
- Ascending Social club
- Descending Order
This method is used to sort a list in place, which means that it mutates it or modifies it straight without creating additional copies, so remember:

You lot volition learn more than nigh mutation in this commodity (I hope!), merely for now information technology'south very of import that yous know that the sort()
method modifies the list, so its original version is lost.
Considering of this, you should only use this method if:
- Y'all want to change (sort) the listing permanently.
- You don't need to continue the original version of the list.
If this fits your needs, then the .sort()
method is exactly what you are looking for.
🔸 Syntax and Arguments
Let's come across how y'all can call .sort()
to accept advantage of its full power.
This is the most basic telephone call (with no arguments):
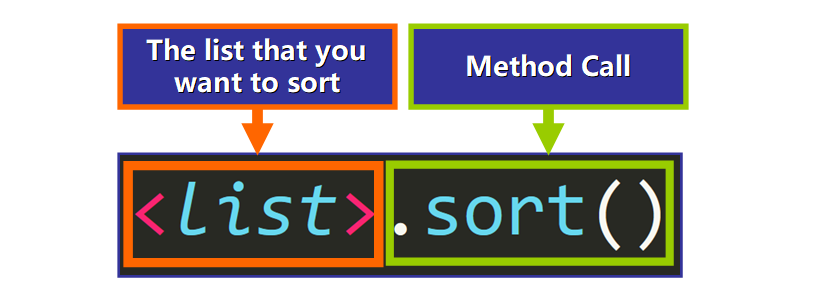
If you don't pass any arguments, by default:
- The listing will exist sorted in ascending order.
- The elements of the listing volition be compared directly using their values with the
<
operator.
For example:
>>> b = [six, iii, 8, 2, seven, 3, ix] >>> b.sort() >>> b [2, 3, 3, 6, vii, 8, ix] # Sorted!
Custom Arguments
To customize how the sort()
method works, yous can pass ii optional arguments:
- Key
- Opposite
Let'south come across how they change the beliefs of this method. Here we take a method call with these 2 arguments:

Before explaining how they work, I would like to explain something that y'all probably noticed in the diagram in a higher place – in the method phone call, the names of the parameters have to exist included before their respective values, like this:
-
key=<f>
-
reverse=<value>
This is because they are keyword-only arguments. If y'all are passing a custom value for them, their names have to be specified in the method telephone call, followed by an equal sign =
and their corresponding values, like this:

Otherwise, if you endeavour to laissez passer the arguments directly as nosotros normally exercise for positional parameters, y'all will see this error considering the function will non know which statement corresponds to which parameter:
TypeError: sort() takes no positional arguments
Reverse
Now that you know what keyword-simply arguments are, let's start with reverse
.
The value of reverse
tin be either Truthful
or Imitation
:
-
Fake
ways that the listing will be sorted in ascending guild. -
Truthful
ways that the list will be sorted in descending (reverse) gild.
💡 Tip: By default, its value is False
– if yous don't pass any arguments for this parameter, the listing is sorted in ascending guild.
Here we take a few examples:
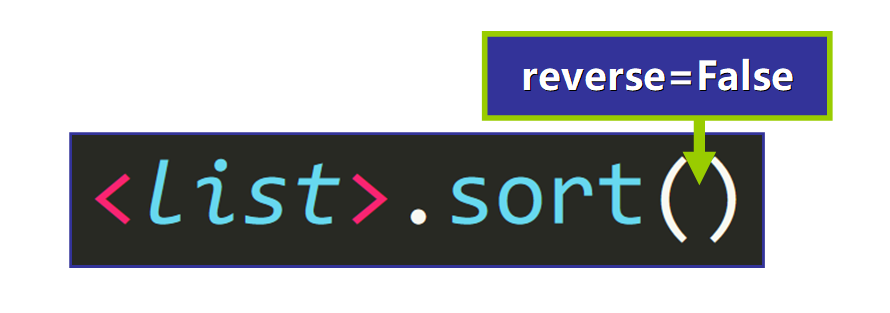
# List of Integers >>> b = [6, iii, 8, ii, 7, three, 9] >>> b.sort() >>> b [2, 3, 3, 6, 7, 8, 9] # Listing of Strings >>> c = ["A", "Z", "D", "T", "U"] >>> c.sort() >>> c ['A', 'D', 'T', 'U', 'Z']
💡 Tip: If the elements of the list are strings, they are sorted alphabetically.

# List of Integers >>> b = [vi, 3, 8, 2, vii, iii, 9] >>> b.sort(reverse=True) >>> b [ix, 8, 7, half-dozen, three, three, 2] # List of Strings >>> c = ["A", "Z", "D", "T", "U"] >>> c.sort(reverse=True) >>> c ['Z', 'U', 'T', 'D', 'A']
💡 Tip: Notice how the list is sorted in descending order if reverse
is True
.
Key
Now that you know how to piece of work with the contrary
parameter, let's see the fundamental
parameter.
This parameter is a niggling flake more detailed because it determines how the elements of the list are be compared during the sorting procedure.

The value of key
is either:
-
None
, which ways that the elements of the list will be compared directly. For example, in a list of integers, the integers themselves can be used for the comparing. - A role of ane argument that generates an intermediate value for each element. This intermediate value is calculated only in one case and it's used to make the comparisons during the entire sorting process. We use this when nosotros don't desire to compare the elements direct, for example, when we want to compare strings based on their length (the intermediate value).
💡 Tip: By default, the value of key
is None
, so the elements are compared direct.
For example:
Let's say that we want to sort a listing of strings based on their length, from the shortest string to the longest cord. Nosotros can pass the part len
equally the value of key
, similar this:
>>> d = ["aaa", "bb", "c"] >>> d.sort(key=len) >>> d ['c', 'bb', 'aaa']
💡 Tip: Notice that nosotros are only passing the name of the function (len
) without parenthesis because nosotros are not calling the function. This is very important.
Notice the divergence betwixt comparing the elements direct and comparison their length (see below). Using the default value of fundamental
(None
) would have sorted the strings alphabetically (left), simply now we are sorting them based on their length (right):

What happens behind the scenes? Each element is passed as an argument to the len()
function, and the value returned by this function telephone call is used to perform the comparisons during the sorting process:
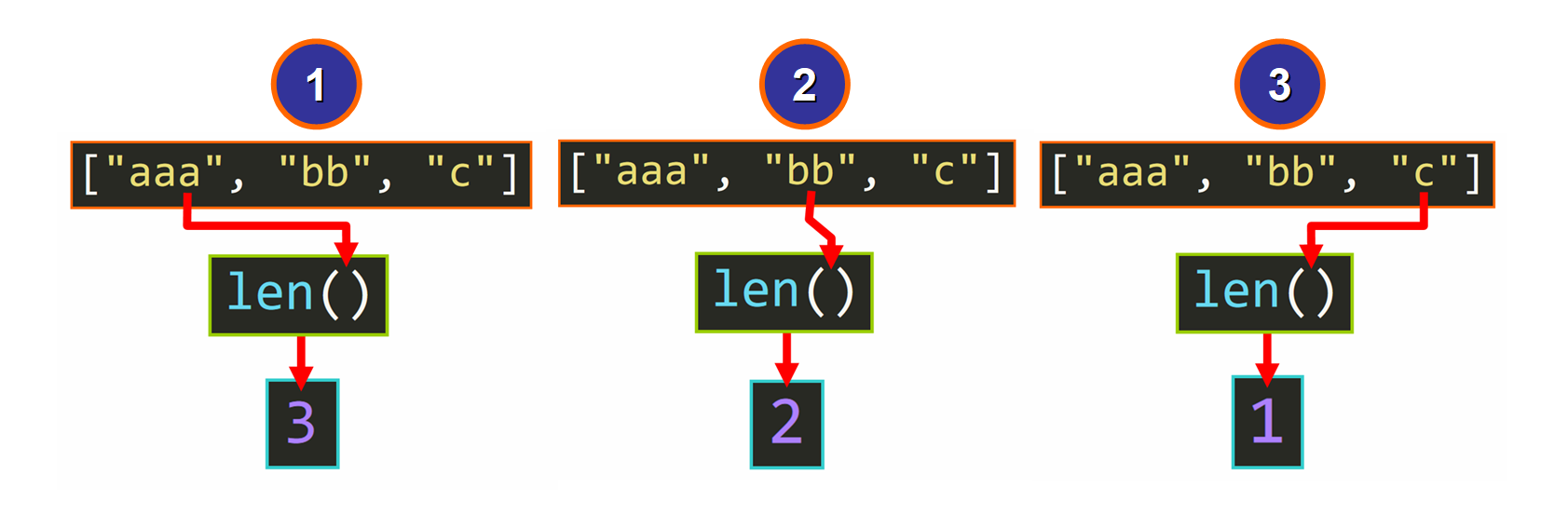
This results in a list with a different sorting criteria: length.
Here we have another example:
Another interesting example is sorting a list of strings as if they were all written in lowercase letters (for example, making "Aa" equivalent to "aa").
According to lexicographical order, capital letters come up earlier lowercase letters:
>>> "East" < "e" True
So the string "Emma"
would come before "emily"
in a sorted listing, even if their lowercase versions would be in the opposite order:
>>> "Emma" < "emily" True >>> "emma" < "emily" False
To avoid distinguishing between capital and lowercase letters, nosotros can pass the function str.lower
every bit central
. This will generate a lowercase version of the strings that will be used for the comparisons:
>>> e = ["Emma", "emily", "Amy", "Jason"] >>> due east.sort(fundamental=str.lower) >>> eastward ['Amy', 'emily', 'Emma', 'Jason']
Notice that now, "emily"
comes earlier "Emma"
in the sorted listing, which is exactly what nosotros wanted.
💡 Tip: if we had used the default sorting procedure, all the strings that started with an uppercase letter would have come before all the strings that started with a lowercase letter:
>>> eastward = ["Emma", "emily", "Amy", "Jason"] >>> due east.sort() >>> e ['Amy', 'Emma', 'Jason', 'emily']
Here is an example using Object-Oriented Programming (OOP):
If nosotros have this very simple Python class:
>>> class Customer: def __init__(self, age): cocky.age = historic period
And nosotros create four instances:
>>> client1 = Client(67) >>> client2 = Client(23) >>> client3 = Client(13) >>> client4 = Client(35)
We can brand a listing that references them:
>>> clients = [client1, client2, client3, client4]
Then, if we define a part to get the age
of these instances:
>>> def get_age(client): return client.age
We tin can sort the list based on their age by passing the get_age
function an an statement:
>>> clients.sort(key=get_age)
This is the final, sorted version of the listing. Nosotros use a for loop to print the age of the instances in the society that they appear in the list:
>>> for client in clients: print(client.age) 13 23 35 67
Exactly what nosotros wanted – now the listing is sorted in ascending order based on the age of the instances.
💡 Tip: Instead of defining a get_age
office, we could take used a lambda function to get the age of each instance, similar this:
>>> clients.sort(key=lambda x: x.age)
Lambda functions are small and simple anonymous functions, which ways that they don't have a proper name. They are very helpful for these scenarios when we only desire to use them in particular places for a very short period of fourth dimension.
This is the bones structure of the lambda function that we are using to sort the list:
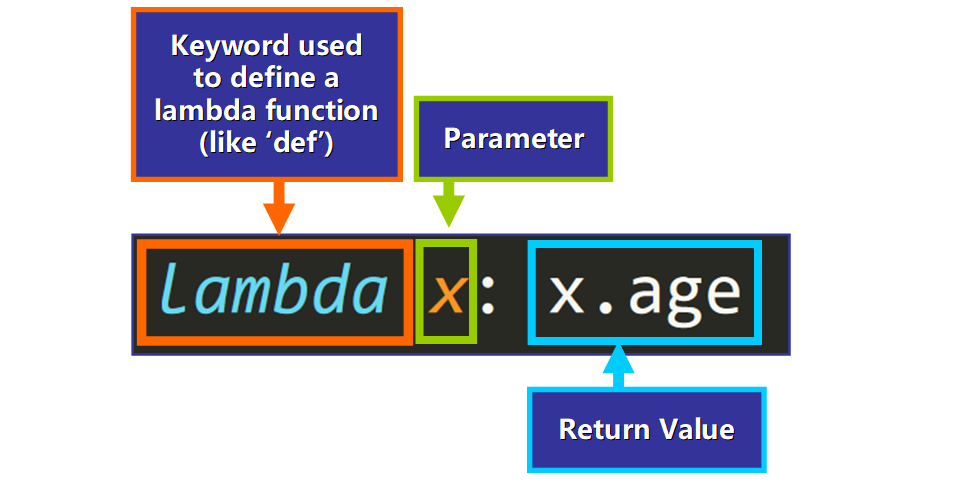
Passing Both Arguments
Awesome! Now you know to customize the functionality of the sort()
method. But you can take your skills to a whole new level by combining the upshot of key
and reverse
in the same method call:
>>> f = ["A", "a", "B", "b", "C", "c"] >>> f.sort(key=str.lower, opposite=Truthful) >>> f ['C', 'c', 'B', 'b', 'A', 'a']
These are the different combinations of the arguments and their effect:

The Order of Keyword-Simply Arguments Doesn't Matter
Since we are specifying the names of the arguments, we already know which value corresponds to which parameter, so we can include either key
or reverse
first in the list and the event will be exactly the same.
So this method call:

Is equivalent to:

This is an instance:
>>> a = ["Zz", "c", "y", "o", "F"] >>> a.sort(key=str.lower, reverse=Truthful) >>> a ['Zz', 'y', 'o', 'F', 'c']
If we change the club of the arguments, we go the exact aforementioned result:
>>> a = ["Zz", "c", "y", "o", "F"] >>> a.sort(reverse=True, key=str.lower) >>> a ['Zz', 'y', 'o', 'F', 'c']
🔹 Return Value
Now let's talk a little flake most the return value of this method. The sort()
method returns None
– information technology does not return a sorted version of the listing, like we might intuitively expect.
According to the Python Documentation:
To remind users that information technology operates by side upshot, information technology does non render the sorted sequence.
Basically, this is used to remind us that we are modifying the original list in retentivity, not generating a new re-create of the list.
This is an case of the render value of sort()
:
>>> nums = [6.5, two.4, vii.3, 3.five, 2.six, vii.four] # Assign the render value to this variable: >>> val = nums.sort() # Cheque the render value: >>> print(val) None
Run across? None
was returned by the method call.
💡 Tip: It is very of import non to confuse the sort()
method with the sorted()
function, which is a function that works very similarly, but doesn't modify the original listing. Instead sorted()
generates and returns a new re-create of the list, already sorted.
This is an example that nosotros tin can use to compare them:
# The sort() method returns None >>> nums = [6.v, 2.4, seven.3, 3.5, 2.six, 7.4] >>> val = nums.sort() >>> print(val) None
# sorted() returns a new sorted copy of the original list >>> nums = [6.5, 2.4, 7.3, iii.5, 2.6, 7.4] >>> val = sorted(nums) >>> val [2.four, 2.six, 3.five, 6.5, 7.iii, 7.4] # Simply it doesn't change the original list >>> nums [6.5, 2.iv, 7.3, 3.five, two.6, 7.four]
This is very important because their effect is very different. Using the sort()
method when you intended to use sorted()
can introduce serious bugs into your program because yous might non realize that the listing is existence mutated.
🔸 The sort() Method Performs a Stable Sort
At present let's talk a footling fleck about the characteristics of the sorting algorithm used past sort()
.
This method performs a stable sort because it works with an implementation of TimSort, a very efficient and stable sorting algorithm.
According to the Python Documentation:
A sort is stable if it guarantees non to alter the relative order of elements that compare equal — this is helpful for sorting in multiple passes (for example, sort by section, then past salary grade).
This means that if ii elements have the same value or intermediate value (key), they are guaranteed to stay in the aforementioned order relative to each other.
Let's run into what I hateful with this. Please take a look at this instance for a few moments:
>>> d = ["BB", "AA", "CC", "A", "B", "AAA", "BBB"] >>> d.sort(key=len) >>> d ['A', 'B', 'BB', 'AA', 'CC', 'AAA', 'BBB']
We are comparing the elements based on their length because we passed the len
function as the statement for key
.
We can run across that there are three elements with length 2: "BB"
, "AA"
, and "CC"
in that order.
Now, notice that these 3 elements are in the same relative social club in the final sorted list:

This is because the algorithm is guaranteed to be stable and the three of them had the same intermediate value (fundamental) during the sorting procedure (their length was 2, so their key was 2).
💡 Tip: The same happened with "A"
and "B"
(length 1) and "AAA"
and "BBB"
(length iii), their original lodge relative to each other was preserved.
At present you know how the sort()
method works, so allow's dive into mutation and how it tin touch on your program.
🔹 Mutation and Risks
As promised, let'southward see how the process of mutation works behind the scenes:
When you define a list in Python, similar this:
a = [1, two, iii, 4]
You create an object at a specific retention location. This location is called the "memory address" of the object, represented past a unique integer called an id.
You tin think of an id as a "tag" used to identify a specific identify in memory:
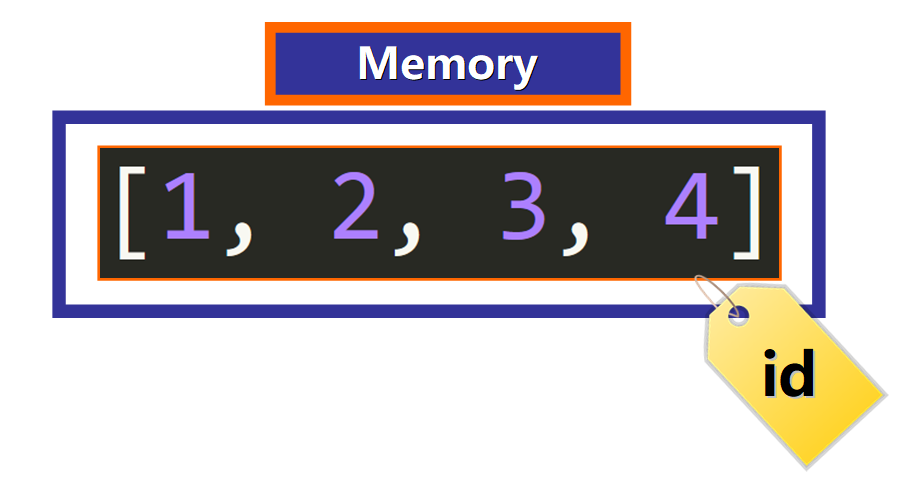
Yous can access a list'south id using the id()
function, passing the list as argument:
>>> a = [1, 2, three, 4] >>> id(a) 60501512
When you mutate the list, you change information technology direct in retention. You may inquire, why is this and then risky?
It's risky considering it affects every unmarried line of code that uses the list subsequently the mutation, so you may be writing lawmaking to work with a list that is completely dissimilar from the actual listing that exists in memory after the mutation.
This is why you need to be very conscientious with methods that cause mutation.
In item, the sort()
method mutates the list. This is an case of its effect:
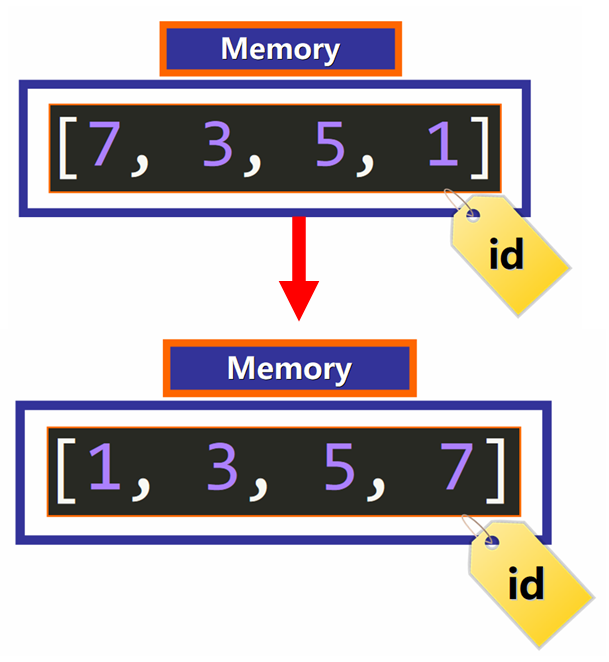
Here is an instance:
# Define a list >>> a = [7, iii, 5, 1] # Check its id >>> id(a) 67091624 # Sort the list using .sort() >>> a.sort() # Check its id (it's the same, so the list is the same object in retention) >>> id(a) 67091624 # Now the list is sorted. It has been mutated! >>> a [1, 3, v, 7]
The list was mutated later calling .sort()
.
Every single line of code that works with list a
afterward the mutation has occurred will utilise the new, sorted version of the listing. If this was non what y'all intended, yous may not realize that other parts of your program are working with the new version of the listing.
Hither is some other example of the risks of mutation inside a function:
# List >>> a = [vii, 3, v, 1] # Role that prints the elements of the list in ascending order. >>> def print_sorted(x): x.sort() for elem in ten: print(elem) # Call the function passing 'a' as argument >>> print_sorted(a) 1 3 5 7 # Oops! The original list was mutated. >>> a [1, three, 5, seven]
The list a
that was passed as argument was mutated, even if that wasn't what you intended when you lot initially wrote the function.
💡 Tip: If a role mutates an argument, information technology should exist conspicuously stated to avoid introducing bugs into other parts of your programme.
🔸 Summary of the sort() Method
- The
sort()
method lets you sort a list in ascending or descending order. - It takes ii keyword-only arguments:
key
andreverse
. -
reverse
determines if the listing is sorted in ascending or descending guild. -
central
is a role that generates an intermediate value for each element, and this value is used to practice the comparisons during the sorting procedure. - The
sort()
method mutates the list, causing permanent changes. You need to be very careful and only use it if you do not demand the original version of the list.
I actually promise that you lot liked my article and found information technology helpful. At present yous can piece of work with the sort()
method in your Python projects. Check out my online courses. Follow me on Twitter. ⭐️
Learn to lawmaking for gratuitous. freeCodeCamp's open source curriculum has helped more than than forty,000 people become jobs every bit developers. Get started
yarbroughsuired97.blogspot.com
Source: https://www.freecodecamp.org/news/the-python-sort-list-array-method-ascending-and-descending-explained-with-examples/
0 Response to "The Data in an Array Can Be Sorted in Either Ascending or Descending Order."
Post a Comment